Push notifications are a powerful tool for enhancing user engagement in mobile applications. With their ability to deliver timely information directly to users, push notifications allow businesses to keep their audience informed, retain users, and increase app activity. However, integrating push notifications into a mobile app can sometimes be a challenging task, especially for cross-platform frameworks like React Native.
In this comprehensive guide, we’ll walk you through everything you need to know about React Native push notifications in 2025, including their architecture, implementation process, and best practices. Whether you’re a beginner or looking to refine your push notification strategy, this guide will cover the essential aspects, from setting up push notifications in React Native to handling background notifications and troubleshooting common issues.
What Are Push Notifications?
Push notifications are messages that are sent to a user’s device from a remote server, even when the app is not actively being used. They appear as alerts, banners, or badges on the user’s device. Push notifications are primarily used for delivering real-time updates, promotions, reminders, and other engaging content to users. For React Native push notifications, the process involves integrating both frontend and backend services to deliver these notifications smoothly across different platforms (iOS and Android).
These notifications are crucial for businesses that want to keep their users engaged and informed about the latest developments, such as new app features, promotions, or activity reminders. By setting up push notification for React Native, you can maintain constant communication with your users and enhance their overall experience.
Related reading:How To Create a PopUp Modal in React Native
Push Notification Architecture in React Native
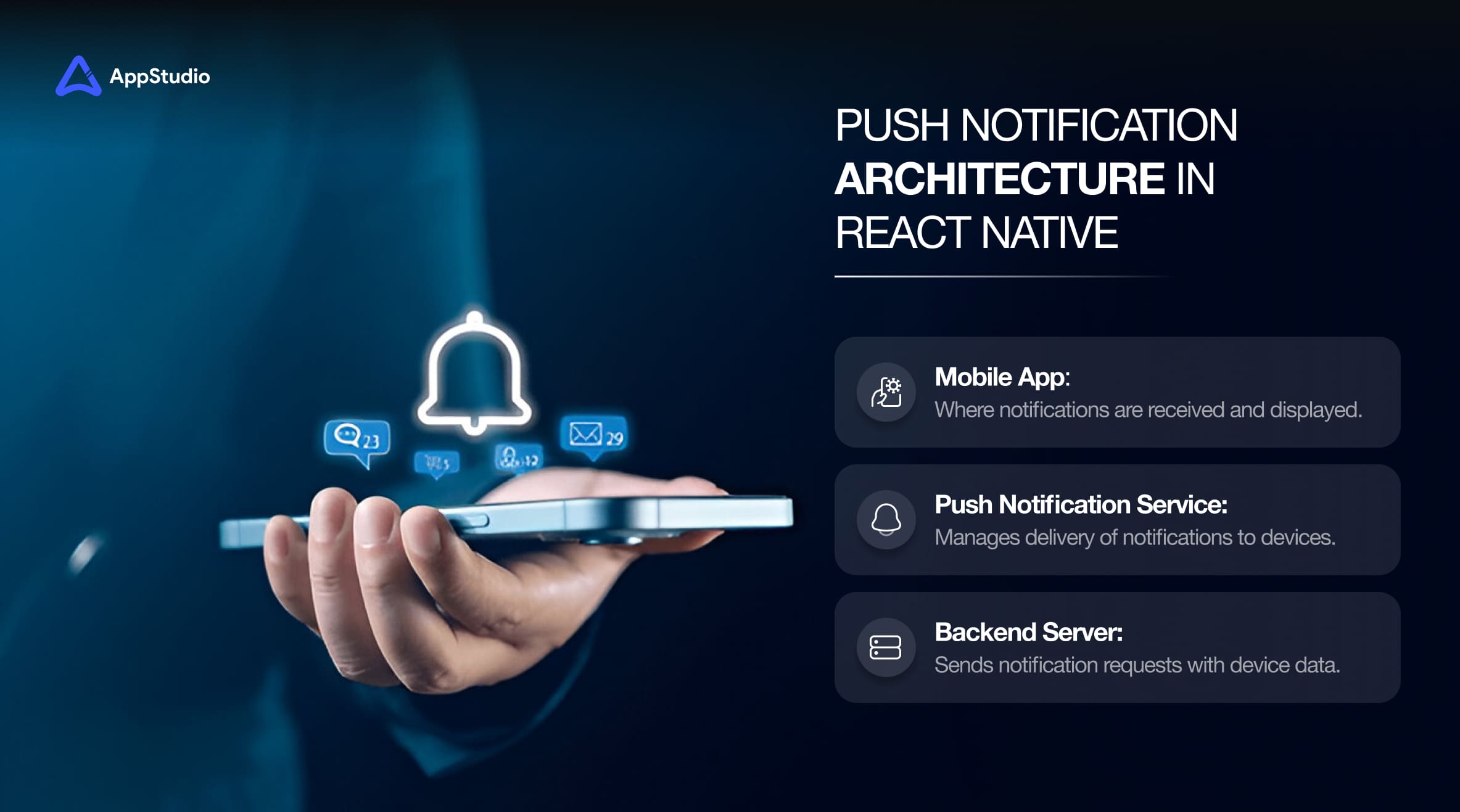
The architecture of React Native push notifications involves three main components: the mobile app, a push notification service, and the backend server.
- Mobile App: This is where notifications are received and displayed. React Native apps must register with the platform’s notification service (FCM for Android, APNs for iOS) to receive push notifications.
- Push Notification Service: This could be Firebase Cloud Messaging (FCM) for Android or Apple Push Notification Service (APNs) for iOS. These services handle the delivery of notifications to the appropriate devices.
- Backend Server: The server sends the push notification request to the push notification service, along with the device’s token, payload (message content), and any other data needed to customize the notification.
This architecture ensures that push notifications on React Native are delivered reliably and efficiently across platforms.
Native Platform-Specific Notification Services (FCM/APNs)
When working with push notification in React Native, developers typically use Firebase Cloud Messaging (FCM) for Android and Apple Push Notification Service (APNs) for iOS. These are the two primary services for delivering notifications to users’ devices on each platform.
- FCM: For Android, FCM is the most widely used service. It provides an easy-to-use and scalable solution for sending notifications. FCM can handle notifications even when the app is in the background or terminated.
- APNs: For iOS, Apple Push Notification Service (APNs) is the native service that handles notification delivery. Unlike FCM, APNs requires additional configuration in Xcode to ensure it works correctly.
These services are critical when considering how to implement push notification in React Native, as they provide the foundation for sending notifications to users’ devices.
Expo Push Notifications and Other Cloud Services
Expo, a popular toolchain for building React Native apps, simplifies the process of setting up push notification in React Native. Expo abstracts away the complexities of dealing with FCM and APNs directly, offering an easier integration for developers, especially for those who may be new to React Native development.
Apart from Expo, cloud services like OneSignal and Pusher provide additional options for handling push notifications on React Native. These services offer advanced features such as targeted notifications, analytics, and even automated campaigns. If you’re looking for a simplified solution, Expo and other cloud services can be excellent alternatives for implementing push notifications.
Other React Native Libraries for Push Notifications
While Expo provides an excellent starting point for integrating notifications, there are other libraries available that offer more control and customization for React Native push notifications. Libraries like Notifee, React Native Firebase, and React Native Push Notification give developers advanced capabilities, such as:
- Notifee: Allows for customized notification appearances and features like media attachments, actions, and buttons.
- React Native Firebase: A robust library that enables integration with Firebase services, including push notifications, analytics, and more.
- React Native Push Notification: A flexible and lightweight solution for managing local and remote notifications in React Native apps.
Choosing the right library depends on your app’s requirements—whether you need simple notification handling or advanced customizations.
Demonstrating How to Set Up Push Notifications in React Native
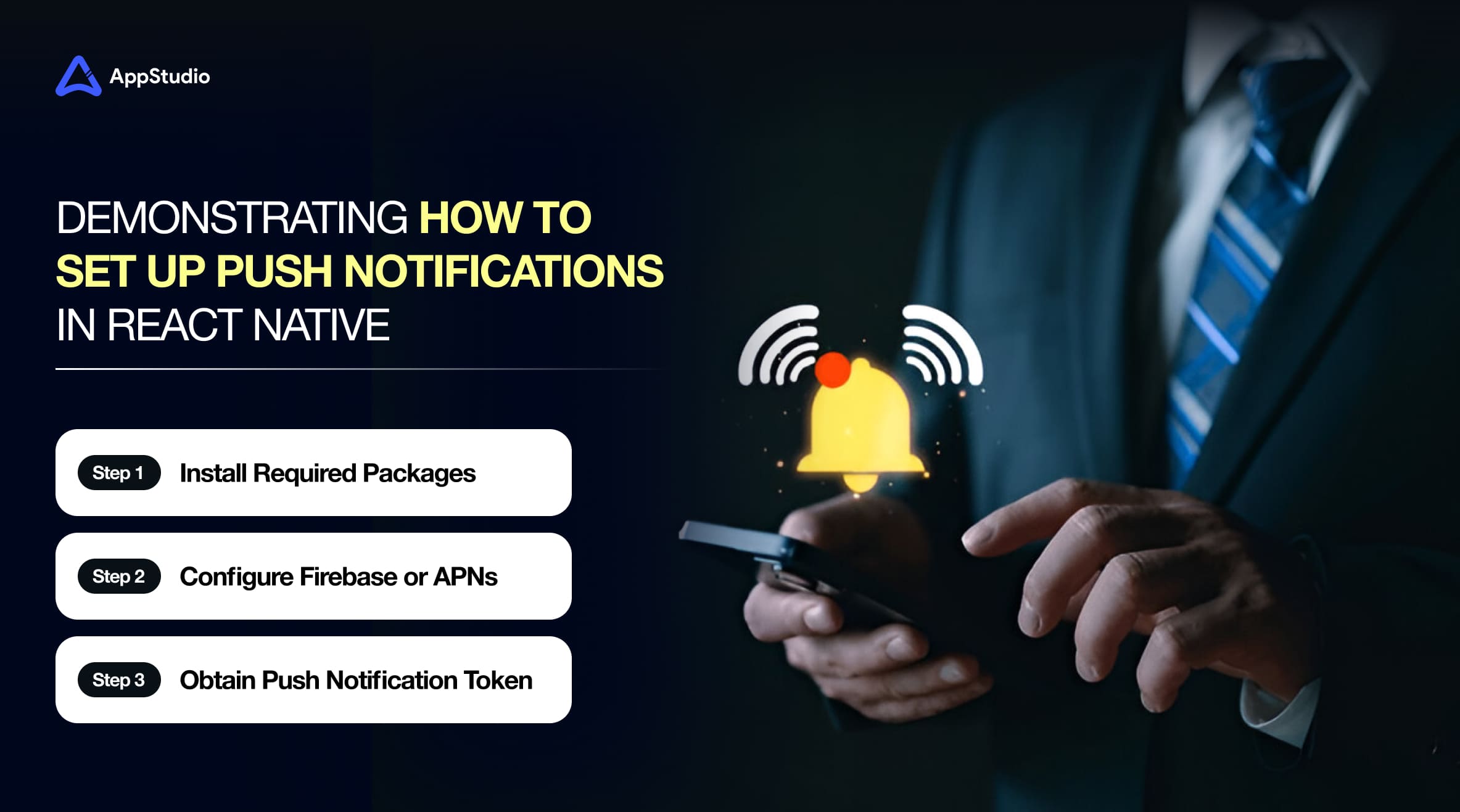
Setting up push notifications in React Native can be broken down into several key steps:
- Install Required Packages: To start implementing push notifications on React Native, you need to install the relevant libraries (e.g., react-native-push-notification, @react-native-firebase/messaging).
- Configure Firebase or APNs: If you are using FCM for Android or APNs for iOS, you need to set up your project in the Firebase Console or Apple Developer portal. This includes generating the appropriate credentials (e.g., Firebase server key or APNs certificates).
- Obtain Push Notification Token: Each device must register for push notifications and receive a token. This token uniquely identifies the device, allowing you to send notifications to it.
Related reading: Top 7 Mistakes to Avoid While Developing React Native Apps
Getting a Push Notification Token
In React Native push notifications, getting the push notification token is essential. This token is used to identify the specific device or app instance that should receive notifications. For example, using React Native Firebase, you can easily retrieve the device token by calling firebase.messaging().getToken().
Once the token is obtained, it should be securely stored in your backend server’s database for future use in sending push notification in React Native.
Sending a Test Notification
Before sending notifications to users, it’s crucial to test the integration. You can do this by sending a test notification from Firebase Console or using tools like Postman to simulate the backend’s request. This will allow you to ensure everything is working as expected and troubleshoot any potential issues early in the development process.
Storing a Push Notification Token in a Project
It’s important to store the device token securely in your backend to manage notifications properly. By associating the token with a user’s account or session, you can target specific users or groups for future notifications.
A typical implementation would involve sending the token to your server after the device registers for notifications, allowing you to manage it as part of your app’s user data.
Sending Notifications on the Server
Once you have stored the device’s token, you can use your backend to send push notifications to the device. This involves making a request to either FCM or APNs, including the token, payload (content of the notification), and any additional data. React Native push notifications will then be sent to the registered device, even if the app is not actively running.
Handling Received Notifications in React Native
Handling notifications is an essential part of how to implement push notification in React Native. When a notification is received, you need to decide how to display it to the user, especially if the app is running in the background or terminated.
You can manage how notifications are displayed and handled in the foreground, background, or when the app is closed. Libraries like Notifee and React Native Firebase offer hooks to manage these events effectively.
Sending Local Notifications with Expo
Expo simplifies the process of sending local notifications within your app. Unlike remote notifications that require server-side interaction, local notifications can be triggered based on user interactions within the app, such as setting reminders or alerts.
Using Expo’s Notifications API, you can schedule notifications locally without needing an external service.
Displaying Notifications with Notifee
For advanced push notifications on React Native, Notifee allows you to create highly customized notifications. This includes adding media, buttons, or custom sounds to notifications. With Notifee, you can deliver a richer notification experience that enhances user engagement.
Background Notifications
Handling background notifications is one of the most challenging aspects of push notification in React Native. Both Android and iOS have strict guidelines for background notifications. By using services like React Native Firebase, you can ensure notifications are delivered even when the app is not actively running, but additional configuration and testing are required to handle these scenarios appropriately.
Which Notifications Library Should You Use?
When choosing which library to use for push notification for React Native, consider the complexity of your app and your specific requirements. If you need simple integration, Expo is an excellent choice. However, for more control and customization, Notifee or React Native Firebase may be better options.
Troubleshooting Common Issues While Setting Up Push Notifications
Setting up React Native push notifications can be tricky, and developers often face issues like incorrect token handling, permission errors, or problems with notifications not appearing in the background. Common troubleshooting steps include checking the server’s configuration, verifying the device’s token, and ensuring the correct permissions are set in the app.
Want to enhance your app with React Native push notifications? Contact AppStudio today to get expert help and boost user engagement with seamless notification integration!
Related reading: A Beginner’s Guide to React Native App Development
Conclusion
Integrating push notifications in React Native is crucial for keeping users engaged and informed in today’s mobile-first world. Whether you choose Expo, React Native Firebase, or other third-party libraries, implementing push notifications on React Native will help you deliver timely updates and improve user experience. By understanding the architecture, setup process, and troubleshooting tips provided in this guide, you can successfully implement push notification for React Native in your app and deliver a seamless, engaging experience to your users.
Frequently Asked Questions
A push notification in React Native is a message sent from a server to a mobile device that alerts the user, even if they are not actively using the app. This notification appears as an alert or banner and can be used to engage users with timely updates, offers, reminders, or other important information. React Native push notifications help keep users informed and engaged with your app in real time.
To implement push notification in React Native, you need to use a combination of React Native libraries (like React Native Firebase or React Native Push Notification), along with a push notification service like Firebase Cloud Messaging (FCM) or Apple Push Notification Service (APNs). The process involves installing the necessary packages, configuring your backend server to send notifications, and handling the device’s push notification token for message delivery. There are also cloud services like Expo Push Notifications that simplify this process for beginners.
When choosing a library for push notification in React Native, you have several options. For simple setups, Expo Push Notifications is a great choice, as it abstracts much of the complexity. If you need more control and customization, React Native Firebase or Notifee offer advanced features like custom sounds, interactive notifications, and background notification handling. React Native Push Notification is another lightweight option for managing local and remote notifications.
FCM (Firebase Cloud Messaging) is primarily used for Android devices, while APNs (Apple Push Notification Service) is used for iOS devices. Both services are essential for React Native push notifications, but the integration process varies. FCM is more straightforward and integrated with Firebase, offering easy-to-use SDKs for Android. In contrast, APNs requires more detailed configuration, particularly with certificates, but is a reliable service for delivering push notifications on iOS devices. You must configure each service individually depending on your target platform when setting up push notification in React Native.
Handling background notifications in React Native requires additional configuration. Both iOS and Android have restrictions for background processes, which means that notifications need to be processed differently depending on the app’s state. Libraries like React Native Firebase offer tools to handle background notifications efficiently. You can configure the app to listen for incoming notifications even when it’s running in the background, allowing your users to stay updated regardless of the app’s activity. When implementing push notification in React Native, it’s important to handle background scenarios to ensure a smooth user experience.
When setting up push notifications on React Native, developers may encounter several common issues such as incorrect token handling, issues with permissions, or problems with notifications not appearing in the background. To troubleshoot, ensure that the device’s token is correctly obtained and registered with the push notification service, and that proper permissions are requested for both iOS and Android. Additionally, if you’re using services like React Native Firebase or Notifee, make sure the configuration and initialization are done correctly for notifications to be sent and received reliably.