In modern mobile app development, managing large datasets effectively is crucial for both performance and user experience. React Native FlatList is a component that helps achieve this by rendering only the items currently visible on the screen, making it an essential tool for handling lists of data. Whether you’re building a social media app, a product listing, or a news feed, FlatList React Native offers an optimized solution that ensures smooth scrolling even with large amounts of data. In this blog, we will explore the core structure of React Native FlatList, how it works, and its key benefits.
What is React Native FlatList?
React Native FlatList is a component used to render large lists of data in a performance-optimized way. Unlike traditional list components that render all items at once, React Native FlatList only renders items that are visible to the user. This dynamic rendering system means that off-screen items are not kept in memory, which greatly improves app performance, especially for long lists. By using React Native FlatList, developers can ensure their apps remain responsive and user-friendly even when handling large datasets.
In addition to performance optimization, FlatList React Native also supports other powerful features like pull-to-refresh, item separators, and infinite scrolling, making it one of the most versatile components for rendering lists.
Related reading:Complete Guide To React Native Push Notifications
7 Key Features of FlatList
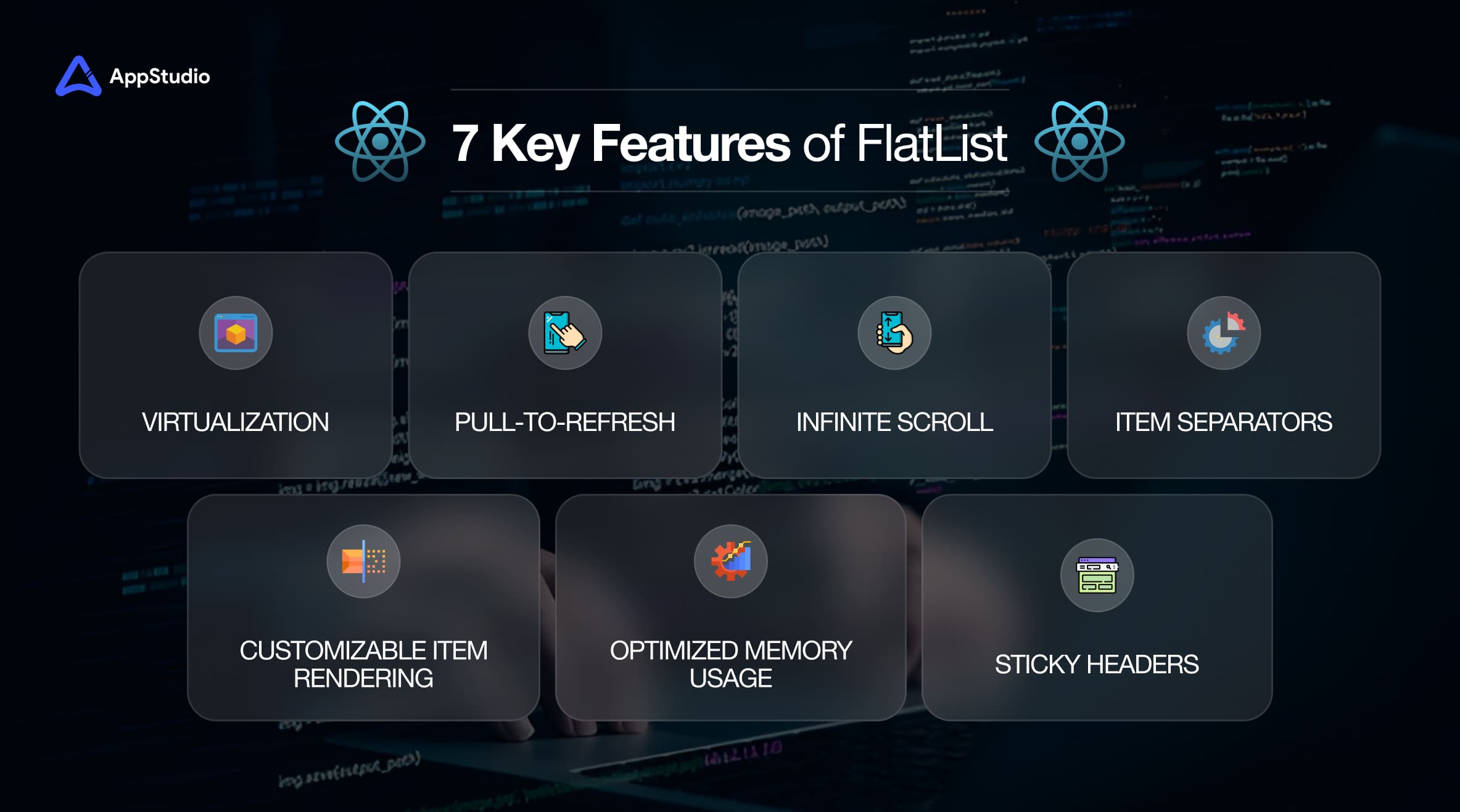
Some of the key features of React Native FlatList include:
- Virtualization: Only the visible items in the list are rendered, improving performance.
- Customizable Item Rendering: The renderItem function allows you to customize how each list item is displayed.
- Infinite Scroll: The onEndReached function allows you to implement infinite scrolling by dynamically loading more items as the user reaches the end of the list.
- Pull-to-Refresh: React Native FlatList supports pull-to-refresh functionality, enabling users to refresh the list content by pulling it downward.
- Item Separators: FlatList lets you add separators between items to improve visual clarity.
- Sticky Headers: FlatList allows you to create sticky headers that stay at the top of the screen as the user scrolls down.
- Optimized Memory Usage: The virtualization process ensures that only visible items are loaded into memory, preventing unnecessary resource consumption.
These features make React Native FlatList a versatile and efficient choice for rendering lists, allowing developers to build fast, interactive, and responsive applications.
How FlatList React Native Works?
The way FlatList React Native works is based on an efficient rendering mechanism called virtualization. Instead of rendering all the items in the list at once, FlatList only renders the items that are currently visible to the user. As the user scrolls through the list, items that scroll out of view are removed from memory, while new items are loaded and displayed.
This virtualization process ensures that even if you have thousands of items in the list, FlatList React Native will only ever render a subset of them at a time, ensuring that your app remains fast and responsive. Additionally, React Native FlatList has built-in support for features like scroll-to-index, sticky headers, and infinite scrolling, making it highly customizable for different use cases.
Understand FlatList Essentials: The Core Structure
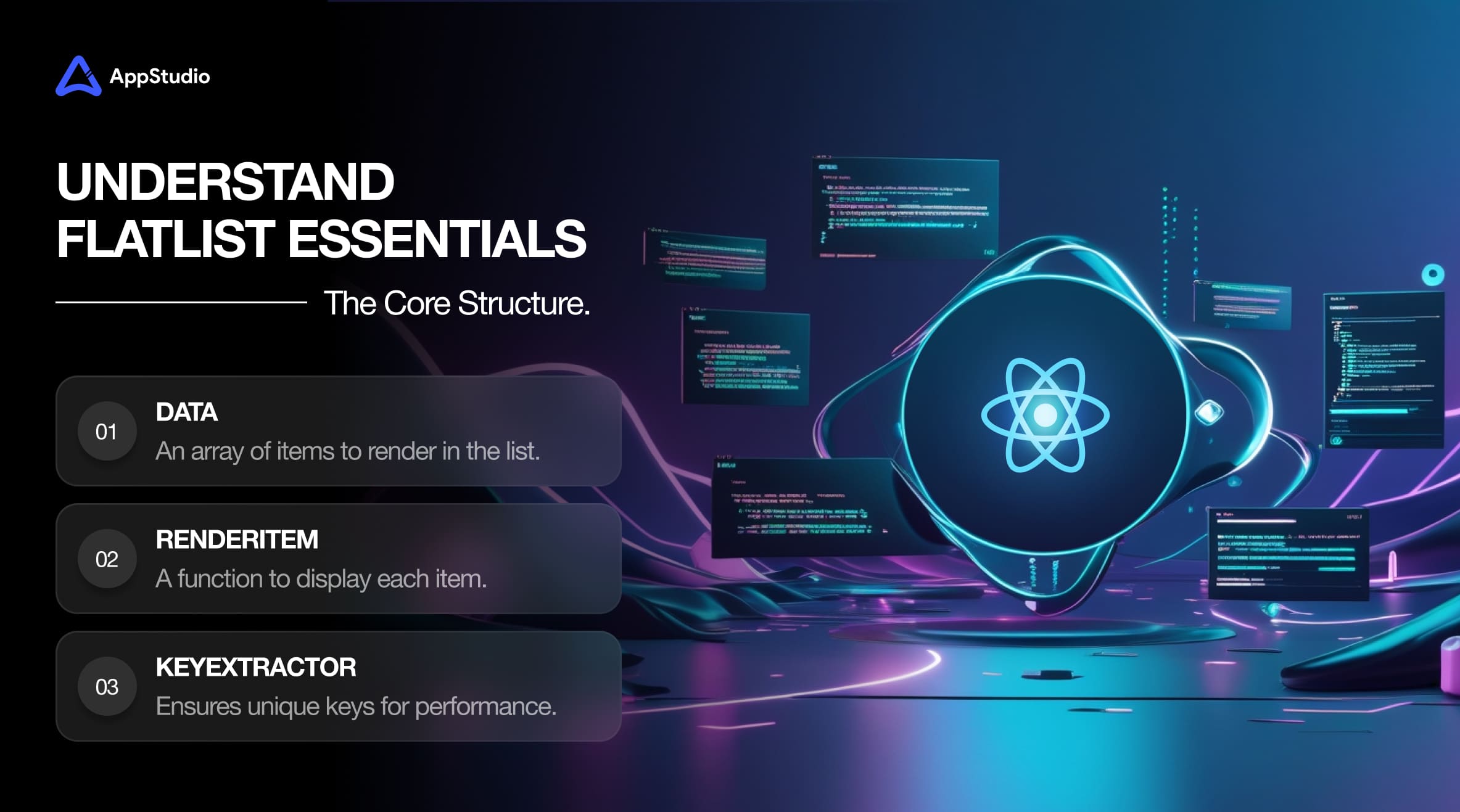
The core structure of React Native FlatList consists of three essential components: the data, the renderItem function, and the keyExtractor.
- Data: The data prop is an array of items that you want to render in the list.
- RenderItem: The renderItem prop is a function used to render each item in the list. It receives an object containing the current item, which you can then customize for display.
- KeyExtractor: The keyExtractor prop ensures that each item in the list has a unique key, which improves the performance when items are added, removed, or reordered.
These components work together to ensure React Native FlatList renders data efficiently by only displaying what is needed, reducing memory usage and increasing responsiveness.
What are the Benefits of Using React Native FlatList?
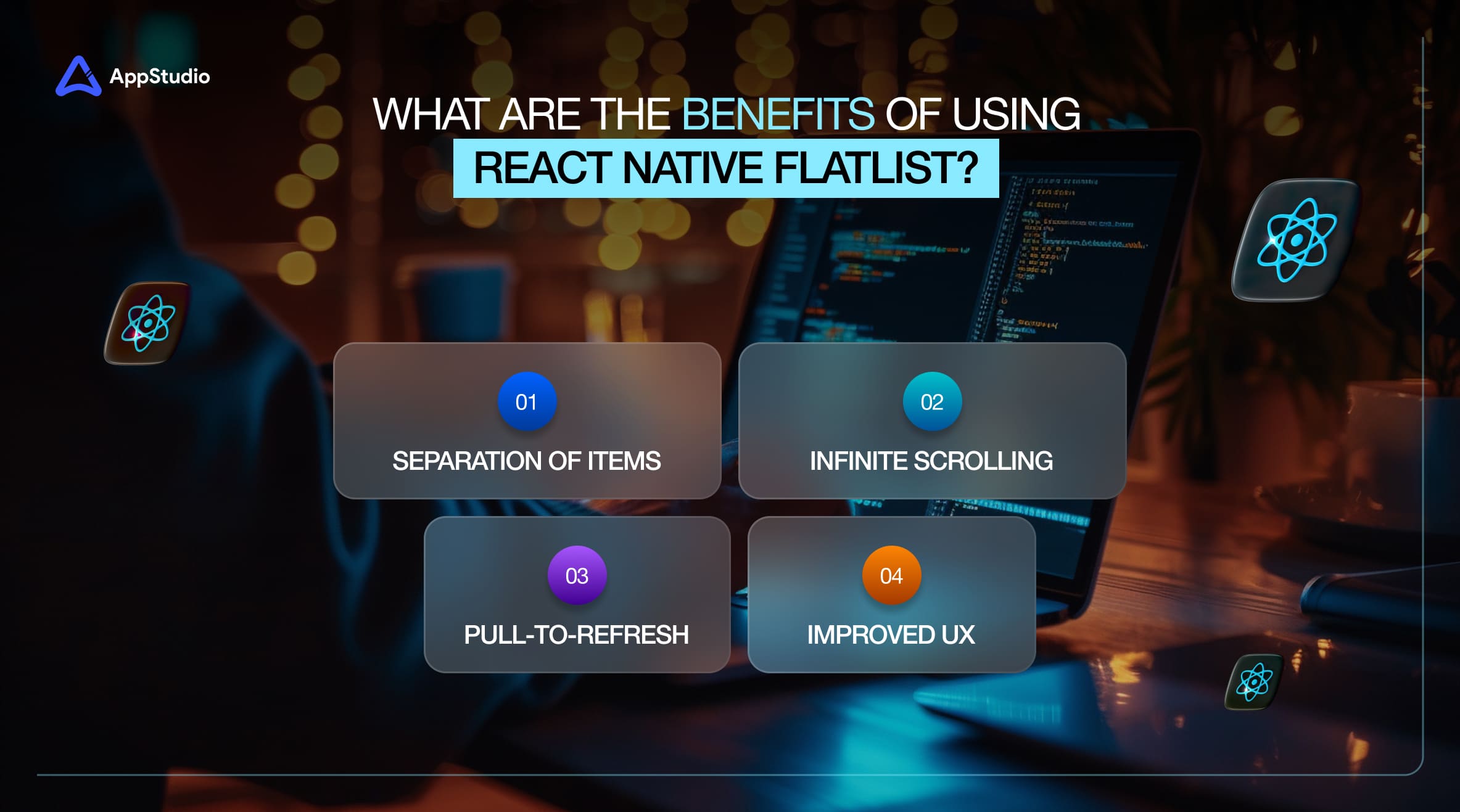
The primary benefit of using React Native FlatList is the performance optimization it offers. By rendering only the visible items in the list, React Native FlatList reduces the memory footprint of your app, making it more responsive and less prone to lag or crashes, especially when dealing with large datasets.
Other benefits include:
- Infinite Scrolling: With the onEndReached prop, FlatList React Native can load more data dynamically as the user scrolls to the end of the list.
- Pull-to-Refresh: The onRefresh prop enables users to refresh the list by pulling down at the top, a common feature in many modern apps.
- Separation of Items: FlatList React Native also allows you to add separators between items, which helps enhance the visual organization of the list.
- Improved UX: The efficient rendering of lists enhances the user experience by providing smooth, uninterrupted scrolling.
Overall, React Native FlatList makes handling large lists more manageable, offering a solution that is both performance-optimized and highly customizable.
Related reading: How To Create a PopUp Modal in React Native
React Native FlatList Best Practices
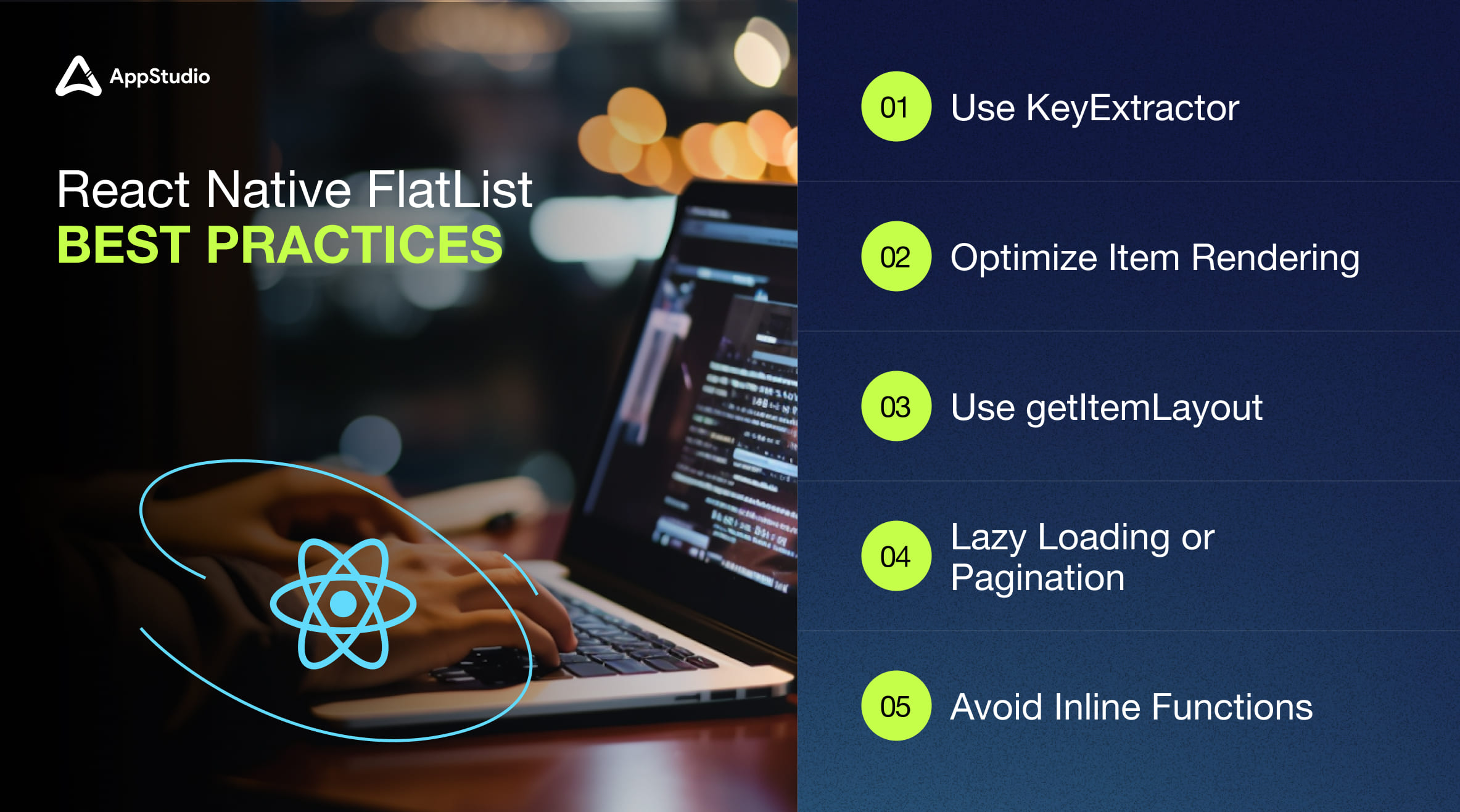
To get the most out of React Native FlatList, it’s essential to follow some best practices to ensure optimal performance and usability. Here are a few React Native FlatList best practices to keep in mind:
Use KeyExtractor
Always provide a keyExtractor function to give each item in the list a unique key. This helps with rendering performance, especially when the list data changes frequently.
Optimize Item Rendering
Use the shouldComponentUpdate or React.memo to optimize item re-rendering. If a list item doesn’t need to be re-rendered every time the list updates, using these techniques can significantly improve performance.
Use getItemLayout
If the items in your list have a fixed height, you can use the getItemLayout prop. This improves performance by calculating the position and size of each item in advance, preventing unnecessary re-measuring.
Lazy Loading or Pagination
When dealing with large datasets, consider using pagination or lazy loading techniques to only load a small portion of the data at a time. React Native FlatList supports infinite scroll out of the box with the onEndReached prop, which triggers a function to load more data as the user scrolls.
Avoid Inline Functions
Inline functions within the renderItem prop can degrade performance. It’s better to define functions outside the render block to reduce unnecessary re-renders.
Use ListHeaderComponent or ListFooterComponent
If you need to display something at the beginning or end of the list (like a header or footer), React Native FlatList provides these props to place components outside of the list content without affecting the list’s scrolling behavior.
By following these React Native FlatList best practices, you can build fast, responsive lists that offer a smooth user experience, even with large datasets.
React Native FlatList Style Example
One of the standout features of React Native FlatList is the ability to customize the appearance of list items. You can use the react native flatlist style prop to style the list and individual items in various ways. Whether you’re looking to change the font, background color, or layout of each item, FlatList provides numerous options for styling.
Here’s a simple react native flatlist style example where we create a list of items with custom styling:
import React from ‘react’;
import { FlatList, Text, View, StyleSheet } from ‘react-native’;
const data = [
{ id: ‘1’, name: ‘Item 1’ },
{ id: ‘2’, name: ‘Item 2’ },
{ id: ‘3’, name: ‘Item 3’ },
];
const App = () => {
return (
<FlatList
data={data}
renderItem={({ item }) => (
<View style={styles.item}>
<Text style={styles.text}>{item.name}</Text>
</View>
)}
keyExtractor={item => item.id}
/>
);
};
const styles = StyleSheet.create({
item: {
padding: 20,
marginVertical: 10,
backgroundColor: ‘#f9f9f9’,
borderRadius: 5,
},
text: {
fontSize: 18,
color: ‘#333’,
},
});
export default App;
In this react native flatlist style example, we’ve customized the list items with padding, margins, and background color. Each item’s text is also styled with font size and color, demonstrating how to use React Native FlatList style to modify the appearance.
Related reading:A Beginner’s Guide to React Native App Development
Conclusion:
React Native FlatList is a powerful component designed to efficiently render large lists of data by only displaying what is necessary at any given time. Whether you need to create a feed, product list, or any other type of content-heavy interface, FlatList React Native provides a performant solution. By using react native flatlist style for custom designs and following React Native FlatList best practices, you can build seamless and high-performance list views. Additionally, integrating React Native FlatList navigation helps make your app more interactive, enhancing the user experience. This combination of performance, customization, and interactivity makes React Native FlatList an essential tool for developers working with large datasets in mobile applications.
Want to enhance your mobile app’s performance with React Native FlatList? Let AppStudio help you build efficient and engaging lists in your app. Contact us today to get started!
Frequently Asked Questions
React Native FlatList is a component used to render large datasets efficiently by only displaying the items that are currently visible on the screen. It optimizes memory usage and performance by dynamically rendering items as they come into view, making it an ideal solution for apps that need to display long lists. React Native FlatList is particularly useful in scenarios where you are working with thousands of items, such as social media feeds, product listings, and news apps.
FlatList React Native works by using a technique called virtualization. It renders only the items currently visible in the user’s viewport, and as the user scrolls, it removes off-screen items from memory and loads new ones. This reduces the overall memory footprint and ensures smoother scrolling, even with large data sets. By not rendering all list items at once, React Native FlatList significantly improves performance and user experience.
When styling React Native FlatList, the most effective approach is to use the style prop and customize individual items with the renderItem function. You can apply custom styles to the list, such as spacing, colors, and fonts, to make it fit the design of your app. A React Native FlatList style example might involve defining padding, margins, background colors, or even customizing fonts for each item. This level of customization allows developers to create lists that align perfectly with the overall app design.
Some React Native FlatList best practices include:
- Always provide a unique keyExtractor to ensure proper item identification and efficient re-renders.
- Use the getItemLayout prop for fixed-height items to avoid unnecessary re-measuring during scroll.
- Use onEndReached for implementing infinite scrolling to load more items as the user scrolls down.
- Avoid inline functions in the renderItem prop for better performance.
- Use React Native FlatList’s ListHeaderComponent and ListFooterComponent props to add headers or footers without impacting list performance.
Following these React Native FlatList best practices ensures that the component is used efficiently, leading to improved app performance and smoother user interactions.
Integrating FlatList React Native navigation into your app allows users to interact with list items and navigate to other parts of the app. By wrapping list items with TouchableOpacity or Pressable components, you can handle taps or clicks, and navigate to another screen using React Navigation. For instance, when an item is clicked, it can trigger a navigation function that directs the user to a detailed screen. This makes React Native FlatList not just a display tool, but also an interactive component for seamless user experiences.
The key features of React Native FlatList include:
- Efficient Rendering: Renders only visible items for optimal memory usage.
- Infinite Scrolling: Supports dynamic loading of new data as the user scrolls.
- Pull-to-Refresh: Built-in support for refreshing the list content by pulling down.
- Item Separators: Allows you to add separators between list items for better visual organization.
- Customizable Styles: Offers flexibility to style items and the overall list.
- Sticky Headers: Ability to create headers that stick to the top as you scroll through the list.
These features make FlatList React Native a powerful and versatile tool for rendering lists in mobile applications, ensuring great performance and a seamless user experience.